w7 <<
Previous Next >> c_ex
ANSIC
練習1
1.輸入數字判斷高於平均身高或低於平均身高或處於平均
#include <stdio.h> // 包含標準輸入/輸出頭文件。
void main()
{
float PerHeight; // 宣告一個浮點數變數 'PerHeight' 來儲存人的身高。
printf("輸入人的身高(以厘米為單位):"); // 提示用戶輸入身高(以厘米為單位)。
scanf("%f", &PerHeight); // 讀取並將用戶的輸入存儲在 'PerHeight' 中。
if (PerHeight < 150.0) // 檢查 'PerHeight' 是否小於 150.0。
printf("這個人是矮子。\n"); // 印出一條消息,指示這個人是矮子。
else if ((PerHeight >= 150.0) && (PerHeight < 165.0)) // 檢查 'PerHeight' 是否介於 150.0 和 165.0 之間。
printf("這個人身高屬於平均水平。\n"); // 印出一條消息,指示這個人身高屬於平均水平。
else if ((PerHeight >= 165.0) && (PerHeight <= 195.0)) // 檢查 'PerHeight' 是否介於 165.0 和 195.0 之間。
printf("這個人是較高的。\n"); // 印出一條消息,指示這個人身高較高。
else
printf("身高異常。\n"); // 印出一條消息,指示身高異常。
}
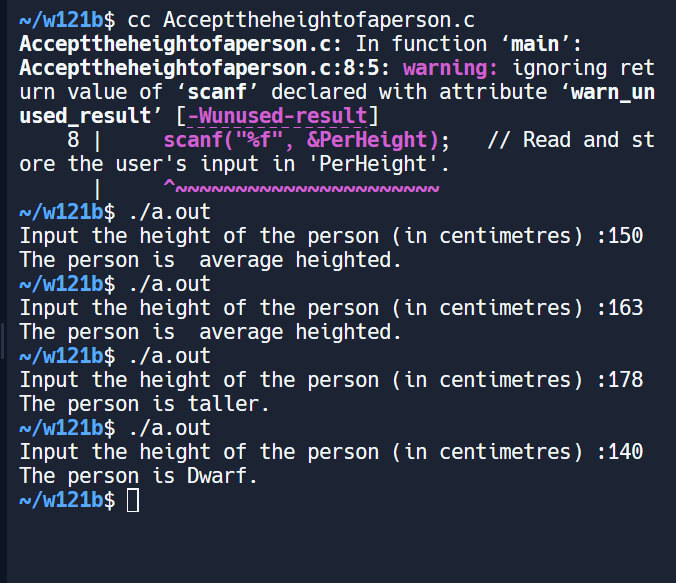
2.輸入攝氏度轉換華氏度
#include <stdio.h> // 包含標準輸入/輸出頭文件。
float temp_f; /* 華氏度 */
float temp_c; /* 攝氏度 */
char line_text[50]; /* 一行輸入 */
int main() {
printf("輸入溫度(攝氏度):"); // 提示用戶輸入攝氏溫度。
fgets(line_text, sizeof(line_text), stdin); // 從用戶讀取一行輸入並存儲在 'line_text' 中。
sscanf(line_text, "%f", &temp_c); // 將 'line_text' 中的輸入轉換為浮點數並存儲在 'temp_c' 中。
temp_f = ((9.0 / 5.0) * temp_c) + 32.0; // 將攝氏溫度轉換為華氏溫度並存儲在 'temp_f' 中。
printf("%f degrees Fahrenheit.\n", temp_f); // 以華氏度打印溫度。
return(0); // 返回0以表示程序成功執行。
}
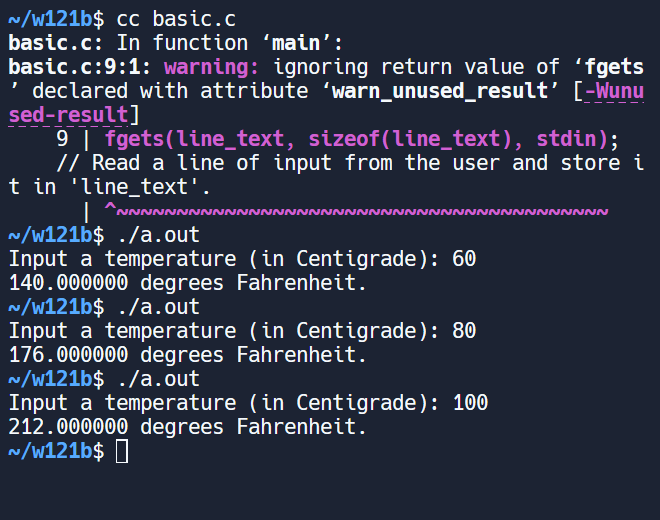
3.用C語言函數獲取當前時間
#define __STDC_WANT_LIB_EXT1__ 1/這一行是一個預處理器指令,用來啟用C標準庫的擴展功能。在這裡,它啟用了擴展函數 ctime_s
#include <time.h>/這裡包含了標準的時間頭文件,其中包含了一些與時間相關的函數和結構。
#include <stdio.h> /這裡包含了標準的輸入/輸出頭文件,以便使用 printf 函數輸出信息。
int main(void)/這是程序的主函數的開始
{
time_t result = time(NULL);/使用 time 函數獲取當前的時間,返回的結果存儲在 result 變數中。
printf("\n%s\n", ctime(&result));/ 使用 ctime 函數將時間轉換為可讀的字符串格式,並通過 printf 函數打印出來。
#ifdef __STDC_LIB_EXT1__/這是一個條件編譯指令,用於檢查是否支持標準庫的擴展功能。
char time_str[26];
ctime_s(time_str,sizeof time_str,&result);/如果支持擴展功能,則使用 ctime_s 函數將時間轉換為可讀的字符串格式,並將結果存儲在 time_str 中。
printf("\n%s\n", time_str);/通過 printf 函數打印使用 ctime_s 轉換後的時間字符串。
#endif
}
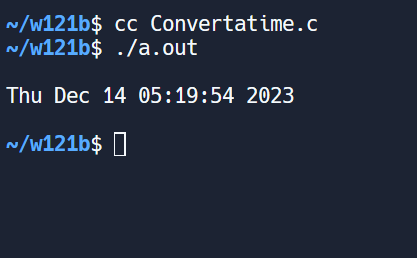
4.用C語言寫一個程式來列印目前日期和時間
#include <time.h>
#include <stdio.h>
#include <stdlib.h>
int main(void)
{
time_t cur_time; // 定義一個 time_t 型變數 cur_time 來存儲當前時間。
char* cur_t_string; // 定義一個指向字符的指針 cur_t_string,用來存儲轉換後的時間字符串。
cur_time = time(NULL); // 使用 time 函數獲取當前時間,並將其存儲在 cur_time 變數中。
if (cur_time == ((time_t)-1))
{
// 如果獲取當前時間失敗,輸出錯誤信息並以失敗的狀態退出程序。
(void) fprintf(stderr, "無法獲取當前日期和時間。\n");
exit(EXIT_FAILURE);
}
cur_t_string = ctime(&cur_time); // 將 cur_time 中的時間轉換為本地時間格式,並將結果存儲在 cur_t_string 中。
if (cur_t_string == NULL)
{
// 如果轉換時間失敗,輸出錯誤信息並以失敗的狀態退出程序。
(void) fprintf(stderr, "無法轉換當前日期和時間。\n");
exit(EXIT_FAILURE);
}
// 通過 printf 函數輸出轉換後的時間字符串。
(void) printf("\n當前時間為:%s\n", cur_t_string);
// 退出程序,表示成功執行。
exit(EXIT_SUCCESS);
}
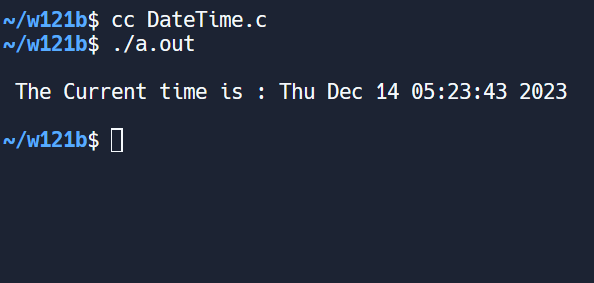
5.輸入名字、姓氏及出生年份
#include <stdio.h> // 包含標準輸入/輸出頭文件。
int main() {
char firstname[20], lastname[20]; // 宣告用於存儲名字和姓氏的字符數組,每個最大大小為20個字符。
int bir_year; // 宣告一個整數變數 'bir_year' 來存儲出生年份。
printf("輸入您的名字:"); // 提示用戶輸入他們的名字。
scanf("%s", firstname); // 讀取並將用戶的輸入存儲在 'firstname' 中。
printf("輸入您的姓氏:"); // 提示用戶輸入他們的姓氏。
scanf("%s", lastname); // 讀取並將用戶的輸入存儲在 'lastname' 中。
printf("輸入您的出生年份:"); // 提示用戶輸入他們的出生年份。
scanf("%d", &bir_year); // 讀取並將用戶的輸入存儲在 'bir_year' 中。
printf("%s %s %d\n", firstname, lastname, bir_year); // 打印名字、姓氏和出生年份。
return 0; // 返回0以表示程序成功執行。
}
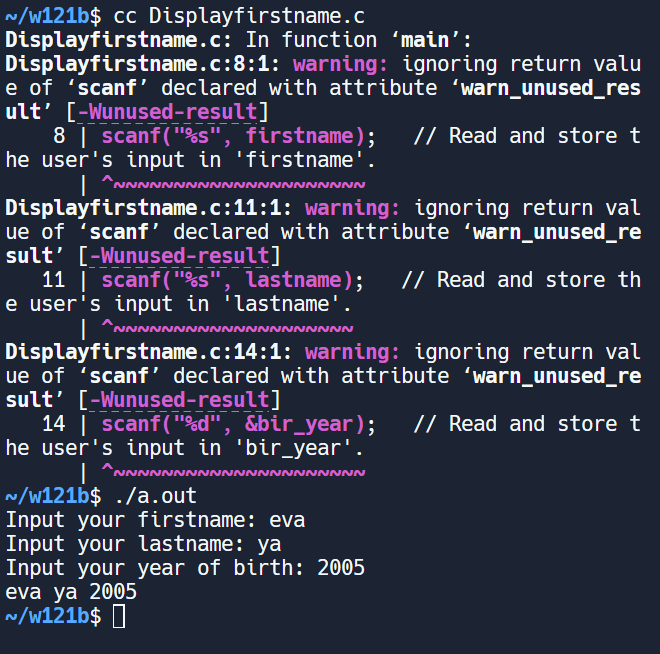
6.檢查數字是否為醜數
# include <stdio.h>
# include <string.h>
int main()
{
int n, x = 0;
printf("\n\n 檢查一個給定數字是否是醜數:\n");
printf("----------------------------------------------------\n");
printf("輸入一個整數數字:");
scanf("%d", &n);
if (n <= 0) {
printf("輸入正確的數字。");
}
while (n != 1)
{
if (n % 5 == 0)
{
n /= 5;
}
else if (n % 3 == 0)
{
n /= 3;
}
else if (n % 2 == 0)
{
n /= 2;
}
else
{
printf("它不是一個醜數。\n");
x = 1;
break;
}
}
if (x == 0)
{
printf("它是一個醜數。\n");
}
return 0;
}
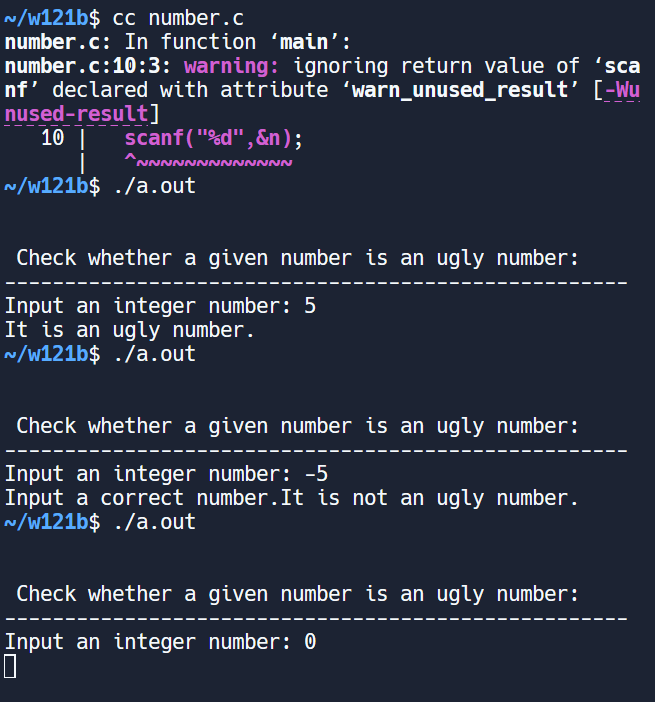
7.將公里每小時轉換為英哩每小時
#include <stdio.h> // 包含標準輸入/輸出頭文件。
float kmph; /* 每小時公里數 */
float miph; /* 每小時英里數(將要計算的) */
char line_text[50]; /* 來自鍵盤的一行輸入 */
int main()
{
printf("輸入每小時公里數:"); // 提示用戶輸入每小時公里數。
fgets(line_text, sizeof(line_text), stdin); // 從用戶讀取一行輸入並將其存儲在 'line_text' 中。
sscanf(line_text, "%f", &kmph); // 將輸入轉換為浮點數並存儲在 'kmph' 中。
miph = (kmph * 0.6213712); // 將每小時公里數轉換為每小時英里數。
printf("%f 英里每小時\n", miph); // 將結果以英里每小時打印出來。
return 0; // 返回0以表示程序成功執行。
}
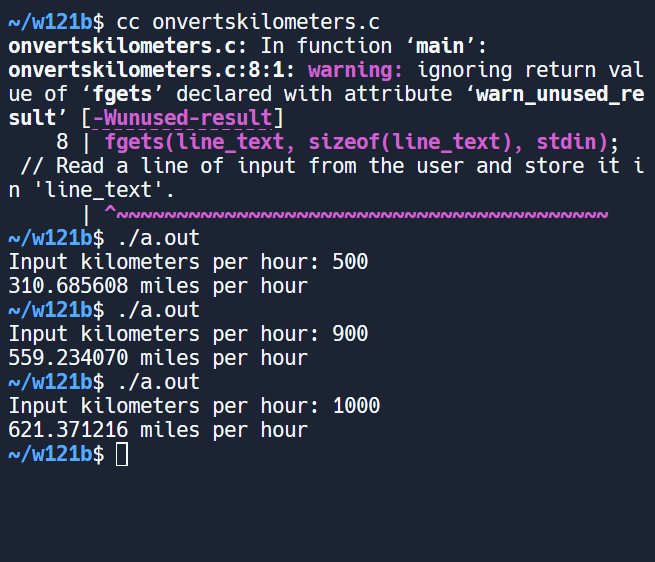
8.顯示兩個整數變量
#include <stdio.h>
void main(void)
{
int m = 10, n, o;
int *z = &m;
printf("\n\n 指針:展示指針的基本聲明:\n");
printf("-------------------------------------------------------\n");
printf(" 這裡有 m=10,n 和 o 是兩個整數變量,*z 是一個整數\n");
printf("\n\n z 存儲 m 的地址 = %p\n", z); // z 是一個指針,所以 %p 會印出地址
printf("\n *z 存儲 m 的值 = %i\n", *z);
printf("\n &m 是 m 的地址 = %p\n", &m); // &m 給出整數變量 m 的地址,所以 %p 是對應的格式符號
printf("\n &n 存儲 n 的地址 = %p\n", &n);
printf("\n &o 存儲 o 的地址 = %p\n", &o);
printf("\n &z 存儲 z 的地址 = %p\n\n", &z); // &z 給出指針 z 的存儲地址,仍然是一個地址,%p 是正確的格式符號
}
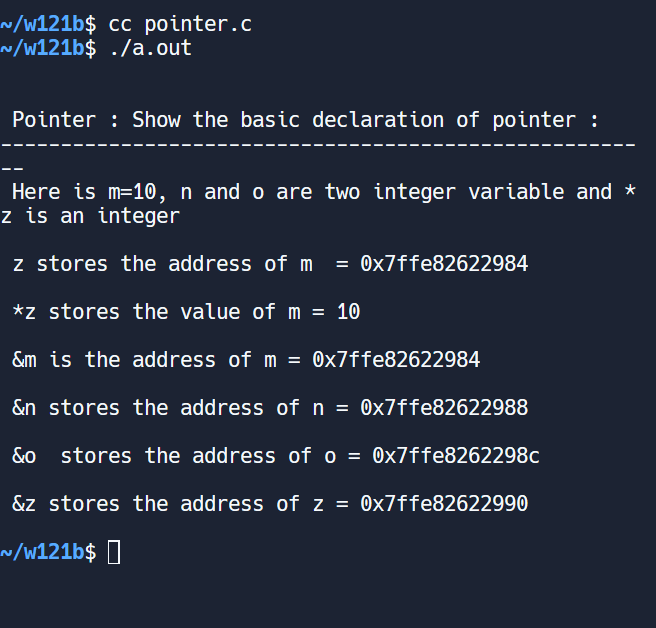
9.輸入1-10的整數查看哪個為正確
#include<stdio.h> // 包含標準輸入/輸出頭文件。
#include<stdlib.h> // 包含標準庫頭文件。
#include<time.h> // 包含時間頭文件,用於生成隨機數。
int main () // 主函數的開始。
{
int number, input; // 宣告兩個整數變數 'number' 和 'input'。
srand ( time(NULL) ); // 使用當前時間初始化隨機種子。
number = rand() % 10 + 1; // 生成一個介於1和10之間的隨機數並存儲在 'number' 中。
do { // 開始一個do-while循環。
printf ("\n猜測數字(1到10):"); // 輸出提示用戶猜測數字的消息。
scanf ("%d", &input); // 讀取用戶的輸入並存儲在 'input' 中。
if (number > input) // 如果隨機數大於用戶的輸入。
printf ("該數字較大\n"); // 輸出消息指示該數字較大。
} while (number != input); // 只要用戶的輸入不等於隨機數,就繼續循環。
printf ("答對了!\n\n"); // 輸出消息指示用戶猜對了。
return 0; // 返回0以表示程序成功執行。
} // 主函數的結尾。
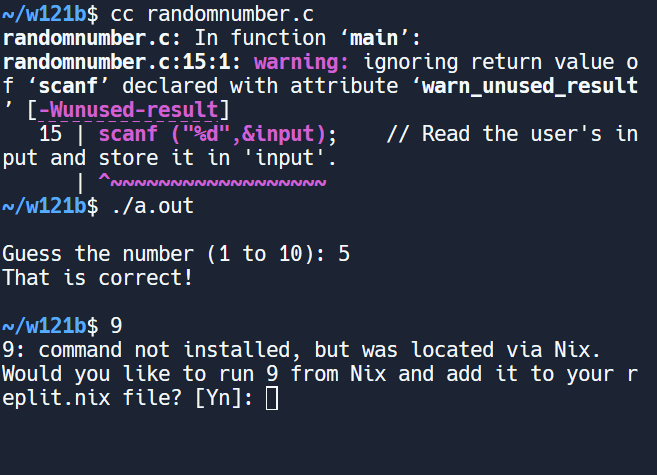
10.輸入任意數的平方
#include <stdio.h>
double square(double num)
{
return (num * num);
}
int main()
{
int num;
double n;
printf("\n\n 函數:計算任意數的平方 :\n");
printf("------------------------------------------------\n");
printf("輸入任意數以計算平方 : ");
scanf("%d", &num);
n = square(num);
printf("%d 的平方是 : %.2f\n", num, n);
return 0;
}
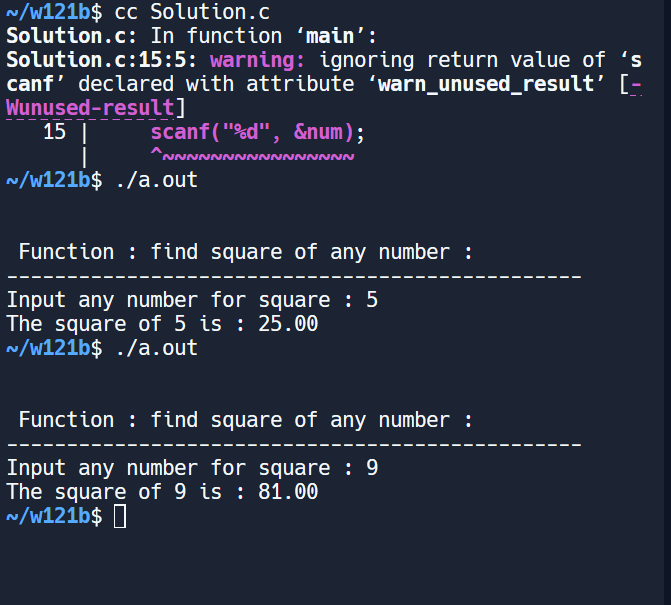
11.用 C 語言編寫程式以建立和顯示單向鏈表
#include <stdio.h>
#include <stdlib.h>
// 單向鏈表中節點的結構
struct node {
int num; // 節點的數據
struct node *nextptr; // 指向下一個節點的地址
} *stnode; // 指向開始節點的指針
// 函數原型
void createNodeList(int n); // 創建鏈表的函數
void displayList(); // 顯示鏈表的函數
// 主函數
int main() {
int n;
// 顯示程序的目的
printf("\n\n 單向鏈表:創建並顯示單向鏈表:\n");
printf("-------------------------------------------------------------\n");
// 輸入鏈表的節點數量
printf(" 輸入節點的數量:");
scanf("%d", &n);
// 創建具有n個節點的鏈表
createNodeList(n);
// 顯示輸入的鏈表數據
printf("\n 鏈表中輸入的數據:\n");
displayList();
return 0;
}
// 函數:創建具有n個節點的鏈表
void createNodeList(int n) {
struct node *fnNode, *tmp;
int num, i;
// 為開始節點分配內存
stnode = (struct node *)malloc(sizeof(struct node));
// 檢查內存分配是否成功
if(stnode == NULL) {
printf(" 無法分配內存。");
} else {
// 從用戶輸入讀取開始節點的數據
printf(" 輸入第1個節點的數據:");
scanf("%d", &num);
stnode->num = num;
stnode->nextptr = NULL; // 將下一個指針設置為NULL
tmp = stnode;
// 創建n個節點並將它們添加到鏈表中
for(i = 2; i <= n; i++) {
fnNode = (struct node *)malloc(sizeof(struct node));
// 檢查內存分配是否成功
if(fnNode == NULL) {
printf(" 無法分配內存。");
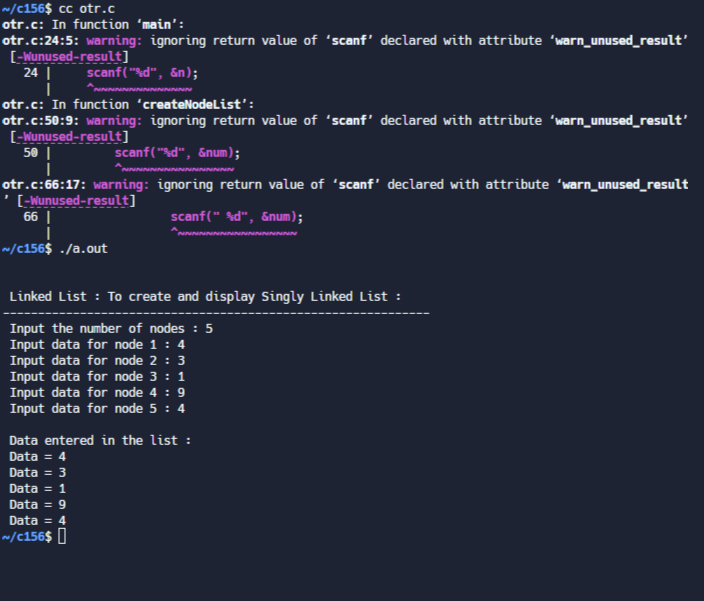
12.實現樹結構的 C 程式
// 包含必要的頭文件
#include <stdio.h>
#include <stdlib.h>
// 二叉樹節點的結構
struct TreeNode {
int data;
struct TreeNode* left;
struct TreeNode* right;
};
// 函數:創建一個新節點
struct TreeNode* createNode(int value) {
// 為一個新的TreeNode分配內存
struct TreeNode* newNode = (struct TreeNode*)malloc(sizeof(struct TreeNode));
// 檢查內存分配是否成功
if (newNode != NULL) {
// 初始化節點數據
newNode->data = value;
newNode->left = NULL;
newNode->right = NULL;
}
// 返回創建的節點
return newNode;
}
// 函數:將節點插入二叉樹
struct TreeNode* insertNode(struct TreeNode* root, int value) {
// 如果樹為空,則創建一個新節點
if (root == NULL) {
return createNode(value);
}
// 如果值小於根的數據,則插入到左子樹中
if (value < root->data) {
root->left = insertNode(root->left, value);
}
// 如果值大於根的數據,則插入到右子樹中
else if (value > root->data) {
root->right = insertNode(root->right, value);
}
// 返回修改後的根
return root;
}
// 函數:執行二叉樹的中序遍歷
void inOrderTraversal(struct TreeNode* root) {
// 中序遍歷樹:左子樹、根、右子樹
if (root != NULL) {
inOrderTraversal(root->left);
printf("%d ", root->data);
inOrderTraversal(root->right);
}
}
// 函數:釋放分配給二叉樹的內存
void freeTree(struct TreeNode* root) {
// 遞歸釋放整棵樹的內存
if (root != NULL) {
freeTree(root->left);
freeTree(root->right);
free(root);
}
}
int main() {
// 初始化二叉樹的根
struct TreeNode* root = NULL;
int nodeValue;
char choice;
// 用戶輸入循環,將節點插入二叉樹
do {
// 提示用戶輸入要插入二叉樹的值
printf("輸入要插入二叉樹的值:");
scanf("%d", &nodeValue);
// 將值插入二叉樹
root = insertNode(root, nodeValue);
// 問用戶是否要插入另一個節點
printf("是否要插入另一個節點?(y/n):");
scanf(" %c", &choice);
} while (choice == 'y' || choice == 'Y');
// 顯示二叉樹的中序遍歷
printf("\n二叉樹的中序遍歷:");
inOrderTraversal(root);
printf("\n");
// 釋放分配給二叉樹的內存
freeTree(root);
// 返回0以表示成功執行
return 0;
}
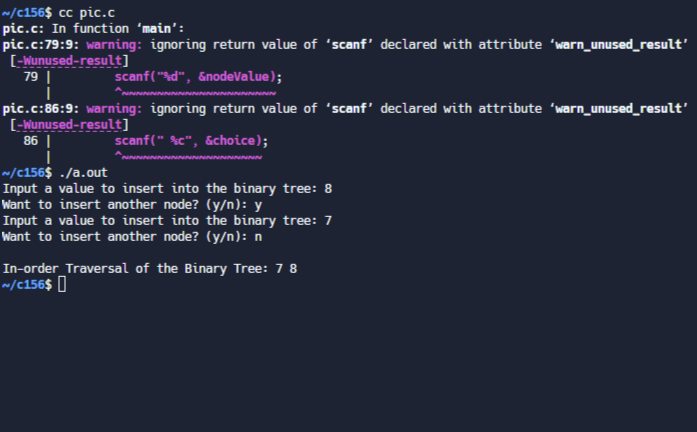
13.實現哈希表的 C 程式
// 包含必要的頭文件
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 10
// 用於鍵值對的結構
struct KeyValuePair {
char key[50];
char value[50];
struct KeyValuePair* next;
};
// 用於哈希表的結構
struct HashTable {
int size;
struct KeyValuePair** table;
};
// 字符串的哈希函數(djb2算法)
unsigned long hashFunction(const char* str) {
unsigned long hash = 5381;
int c;
while ((c = *str++) != '\0') {
hash = ((hash << 5) + hash) + c; // hash * 33 + c
}
return hash;
}
// 函數:創建一個新的鍵值對
struct KeyValuePair* createKeyValuePair(const char* key, const char* value) {
struct KeyValuePair* newPair = (struct KeyValuePair*)malloc(sizeof(struct KeyValuePair));
if (newPair != NULL) {
strcpy(newPair->key, key);
strcpy(newPair->value, value);
newPair->next = NULL;
}
return newPair;
}
// 函數:創建一個新的哈希表
struct HashTable* createHashTable(int size) {
struct HashTable* newTable = (struct HashTable*)malloc(sizeof(struct HashTable));
if (newTable != NULL) {
newTable->size = size;
newTable->table = (struct KeyValuePair**)calloc(size, sizeof(struct KeyValuePair*));
}
return newTable;
}
// 函數:將一個鍵值對插入哈希表
void insert(struct HashTable* hashTable, const char* key, const char* value) {
unsigned long index = hashFunction(key) % hashTable->size;
// 創建一個新的鍵值對
struct KeyValuePair* newPair = createKeyValuePair(key, value);
// 在鏈表的開頭插入新對
newPair->next = hashTable->table[index];
hashTable->table[index] = newPair;
}
// 函數:檢索與鍵關聯的值
const char* retrieve(struct HashTable* hashTable, const char* key) {
unsigned long index = hashFunction(key) % hashTable->size;
struct KeyValuePair* current = hashTable->table[index];
// 遍歷索引處的鏈表
while (current != NULL) {
if (strcmp(current->key, key) == 0) {
return current->value; // 找到鍵,返回值
}
current = current->next;
}
return "未找到鍵"; // 未找到鍵
}
// 函數:顯示哈希表的內容
void displayHashTable(struct HashTable* hashTable) {
for (int i = 0; i < hashTable->size; i++) {
printf("[%d] -> ", i);
struct KeyValuePair* current = hashTable->table[i];
while (current != NULL) {
printf("(%s, %s) -> ", current->key, current->value);
current = current->next;
}
printf("NULL\n");
}
}
// 函數:釋放分配給哈希表的內存
void freeHashTable(struct HashTable* hashTable) {
for (int i = 0; i < hashTable->size; i++) {
struct KeyValuePair* current = hashTable->table[i];
while (current != NULL) {
struct KeyValuePair* temp = current;
current = current->next;
free(temp);
}
}
free(hashTable->table);
free(hashTable);
}
int main() {
struct HashTable* hashTable = createHashTable(TABLE_SIZE);
// 插入鍵值對
insert(hashTable, "Red", "#ff0000");
insert(hashTable, "Green", "008000");
insert(hashTable, "Blue", "#0000FF");
insert(hashTable, "Yellow", "#FFFF00");
insert(hashTable, "Orange", "#FFA500");
printf("初始哈希表:\n");
displayHashTable(hashTable);
printf("\n");
// 檢索並打印特定鍵的值
printf("鍵'Red'的值:%s\n", retrieve(hashTable, "Red"));
printf("鍵'Yellow'的值:%s\n", retrieve(hashTable, "Yellow"));
printf("鍵'White'的值:%s\n", retrieve(hashTable, "White"));
printf("\n");
// 釋放分配的內存
freeHashTable(hashTable);
return 0;
}
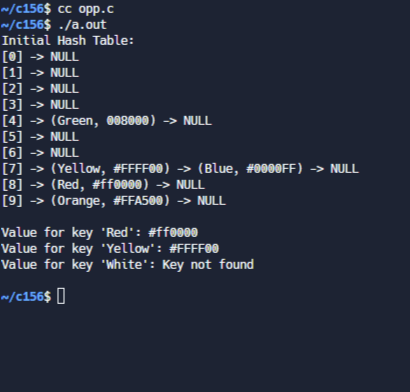
14.C For 迴圈
#include <stdio.h> // 包含標準輸入/輸出頭文件。
int main()
{
int i, j; // 声明用于迭代的變量。
char alph = 'A'; // 初始化一個字符變量為 'A'。
int n, blk; // 声明用戶輸入和區塊計數的變量。
int ctr = 1; // 初始化計數器。
printf("輸入金字塔中字母的數量(小於26):");
scanf("%d", &n); // 提示用戶輸入並將其存儲在變量 'n' 中。
for (i = 1; i <= n; i++) // 外部循環,用於行。
{
for(blk = 1; blk <= n - i; blk++) // 循環以在字母之前打印空格。
printf(" ");
for (j = 0; j <= (ctr / 2); j++) // 循環以按升序打印字母。
{
printf("%c ", alph++);
}
alph = alph - 2; // 在打印一半後減少字符。
for (j = 0; j < (ctr / 2); j++) // 循環以按降序打印字母。
{
printf("%c ", alph--);
}
ctr = ctr + 2; // 為下一行增加計數器。
alph = 'A'; // 將字符重置為 'A' 以供下一行使用。
printf("\n"); // 在打印一行後移到下一行。
}
return 0; // 表示程序已成功執行。
}
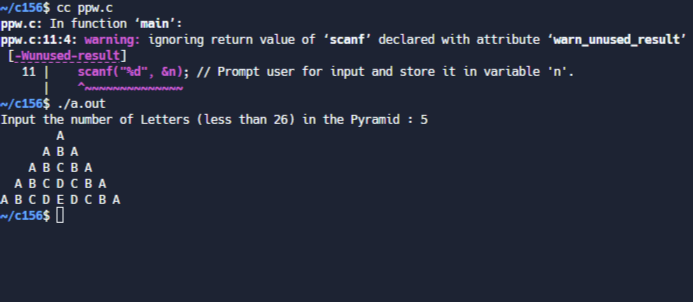
15.編寫一個 C 程式來反轉給定整數的數位
#include <stdio.h>
int reverse(int n) {
int d, y = 0;
while (n) {
d = n % 10;
if ((n > 0 && y > (0x7fffffff - d) / 10) ||
(n < 0 && y < ((signed)0x80000000 - d) / 10)) {
return 0;
}
y = y * 10 + d;
n = n / 10;
}
return y;
}
int main(void)
{
int i = 123;
printf("原始整數: %d ", i);
printf("\n反轉後的整數: %d ", reverse(i));
i = 208478933;
printf("\n原始整數: %d ", i);
printf("\n反轉後的整數: %d ", reverse(i));
i = -73634;
printf("\n原始整數: %d ", i);
printf("\n反轉後的整數: %d ", reverse(i));
return 0;
}
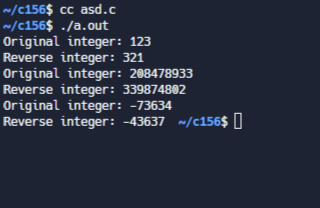
16.C 遞歸
#include<stdio.h>
int term;
int fibonacci(int prNo, int num);
void main()
{
static int prNo = 0, num = 1;
printf("\n\n 遞歸 : 打印斐波那契數列 :\n");
printf("-----------------------------------------\n");
printf(" 輸入要打印的數列項數 (< 20) : ");
scanf("%d", &term);
printf(" 數列為 :\n");
printf(" 1 ");
fibonacci(prNo, num);
printf("\n\n");
}
int fibonacci(int prNo, int num)
{
static int i = 1;
int nxtNo;
if (i == term)
return (0);
else
{
nxtNo = prNo + num;
prNo = num;
num = nxtNo;
printf("%d ", nxtNo);
i++;
fibonacci(prNo, num); // 遞歸,調用 fibonacci 函數本身
}
return (0);
}
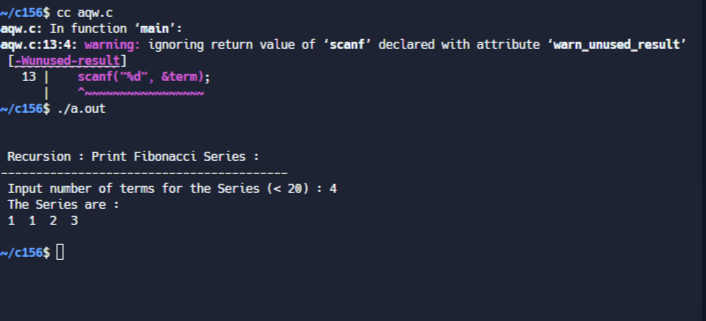
17.編寫一個 C 程式以使用內聯函數計算兩個整數的最小值
#include <stdio.h>
inline int min(int x, int y) {
return (x < y) ? x : y;
}
int main() {
int a = 110, b = 120;
printf("%d 和 %d 的最小值是 %d\n", a, b, min(a, b));
a = -110, b = -120;
printf("%d 和 %d 的最小值是 %d\n", a, b, min(a, b));
return 0;
}
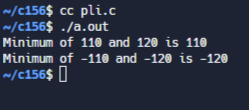
18.創建名為“Employee”的結構來存儲員工詳細資訊,例如員工ID、姓名和工資。編寫程式,輸入三名員工的數據,找到薪水最高的員工,並顯示他們的資訊。
#include <stdio.h>
// 定義結構 "Employee"
struct Employee {
int employeeID;
char name[50];
float salary;
};
int main() {
// 声明變量以存儲三名員工的詳細信息
struct Employee employee1, employee2, employee3;
// 輸入第一名員工的詳細信息
printf("輸入第一名員工的詳細信息:\n");
printf("員工ID: ");
scanf("%d", &employee1.employeeID);
printf("姓名: ");
scanf("%s", employee1.name); // 假設名字不包含空格
printf("薪資: ");
scanf("%f", &employee1.salary);
// 輸入第二名員工的詳細信息
printf("\n輸入第二名員工的詳細信息:\n");
printf("員工ID: ");
scanf("%d", &employee2.employeeID);
printf("姓名: ");
scanf("%s", employee2.name);
printf("薪資: ");
scanf("%f", &employee2.salary);
// 輸入第三名員工的詳細信息
printf("\n輸入第三名員工的詳細信息:\n");
printf("員工ID: ");
scanf("%d", &employee3.employeeID);
printf("姓名: ");
scanf("%s", employee3.name);
printf("薪資: ");
scanf("%f", &employee3.salary);
// 找到薪資最高的員工
struct Employee highestSalaryEmployee;
if (employee1.salary >= employee2.salary && employee1.salary >= employee3.salary) {
highestSalaryEmployee = employee1;
} else if (employee2.salary >= employee1.salary && employee2.salary >= employee3.salary) {
highestSalaryEmployee = employee2;
} else {
highestSalaryEmployee = employee3;
}
// 顯示薪資最高的員工信息
printf("\n薪資最高的員工:\n");
printf("員工ID: %d\n", highestSalaryEmployee.employeeID);
printf("姓名: %s\n", highestSalaryEmployee.name);
printf("薪資: %.2f\n", highestSalaryEmployee.salary);
return 0;
}
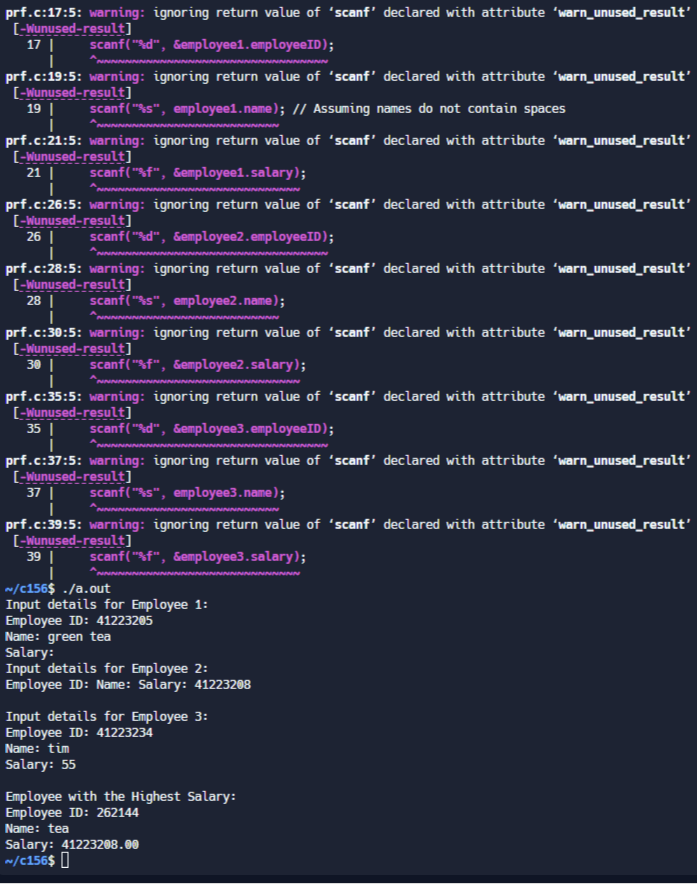
19.編寫一個 C 程式來讀取候選人的年齡並確定他是否有資格投票
#include <stdio.h> // 包含標準輸入/輸出頭文件。
void main()
{
int vote_age; // 声明一個整數變量 'vote_age' 以存儲候選人的年齡。
printf("輸入候選人的年齡 : "); // 提示用戶輸入候選人的年齡。
scanf("%d",&vote_age); // 讀取並將用戶的輸入存儲在 'vote_age' 中。
if (vote_age < 18) // 檢查 'vote_age' 是否小於18。
{
printf("對不起,您無資格投票。\n"); // 印出一條消息,指示候選人無資格投票。
printf("您將在 %d 年後有資格投票。\n", 18 - vote_age); // 印出候選人有資格投票所需的年數。
}
else
printf("恭喜!您有資格投票。\n"); // 印出一條消息,指示候選人有資格投票。
}
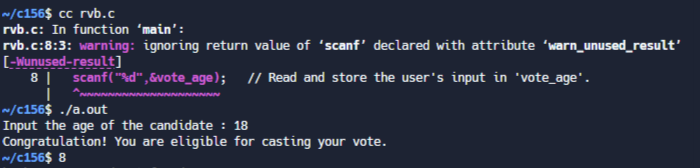
20.編寫一個 C 程式以使用哈希 (#) 列印塊 F,其中 F 的高度為 6 個字元,寬度為 5 個字元和 4 個字元。
#include <stdio.h>
int main()
{
// 打印一行井號
printf("######\n");
// 打印一個單獨的井號
printf("#\n");
// 打印一個單獨的井號
printf("#\n");
// 打印一行井號
printf("#####\n");
// 打印一個單獨的井號
printf("#\n");
// 打印一個單獨的井號
printf("#\n");
// 打印一個單獨的井號
printf("#\n");
return(0);
}
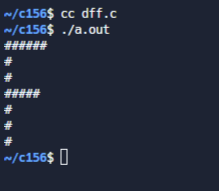
w7 <<
Previous Next >> c_ex