練習一 <<
Previous Next >> w11
練習二
1.將兩個數位相乘
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
/* 乘法計算兩個浮點數 */
int main()
{
float this_is_a_number1, this_is_a_number2, total;
printf("請輸入一個數字:\n ");
scanf("%f", &this_is_a_number1); /* 讀取數字 */
printf("你輸入了 %f\n", this_is_a_number1);
printf("請再輸入一個數字:\n");
scanf("%f", &this_is_a_number2); /* 讀取數字 */
printf("你輸入了 %f\n", this_is_a_number2);
total = this_is_a_number1 * this_is_a_number2; /* 乘法運算 */
printf("兩數的積為 %f\n", total);
return 0;
}
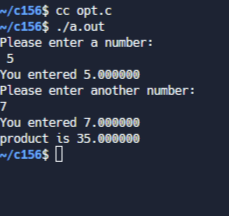
2.數字的總和
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
/* 演示使用 for 迴圈 */
int main()
{
float this_is_a_number, total;
int i;
total = 0;
/* for 迴圈執行 10 次 */
for (i = 0; i < 10; i++)
{
printf("請輸入一個數字:\n ");
scanf("%f", &this_is_a_number); /* 讀取數字 */
total = total + this_is_a_number;
}
printf("總和為 = %f\n", total);
return 0;
}
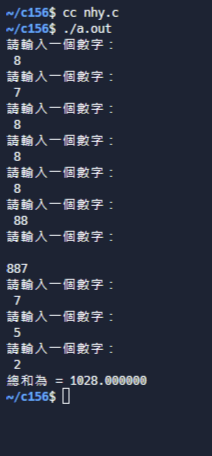
3.判斷數字大小
#include <stdio.h>
/* if 操作的範例 */
int main()
{
int this_is_a_number;
printf("請輸入一個介於1和10之間的整數:\n ");
scanf("%d", &this_is_a_number);
if (this_is_a_number < 6)
printf("這個數字小於6;\n ");
printf("請輸入一個介於10和20之間的整數:\n ");
scanf("%d", &this_is_a_number);
if (this_is_a_number < 16)
printf("這個數字小於16\n ");
else
printf("這個數字大於15\n ");
return 0;
}
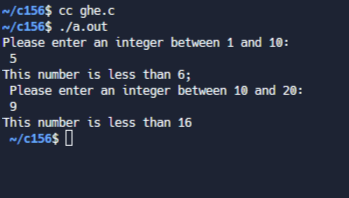
4.陣列
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
/* 展示使用陣列的程式 */
int main()
{
int arr1[8]; /* 定義包含8個整數的陣列 */
int i;
printf("輸入8個整數數字\n");
for (i = 0; i < 8; i++)
{
scanf("%d", &arr1[i]); /* 讀取到 arr1[i] */
}
printf("您的8個數字是\n");
for (i = 0; i < 8; i++)
{
printf("%d ", arr1[i]);
}
printf("\n");
return 0;
}
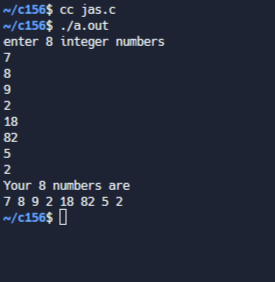
5.sizeof命令
/* 程式示範使用 sizeof 命令 */
#include <stdio.h>
#include <limits.h>
#include <math.h>
int main() {
int sizeofint;
unsigned int sizeofunsint;
float sizeoffloat;
double sizeofdouble;
printf("int 的儲存空間大小:%lu 位元組\n", sizeof(sizeofint));
printf("unsigned int 的儲存空間大小:%lu 位元組\n", sizeof(sizeofunsint));
printf("float 的儲存空間大小:%lu 位元組\n", sizeof(sizeoffloat));
printf("double 的儲存空間大小:%lu 位元組\n", sizeof(sizeofdouble));
return 0;
}
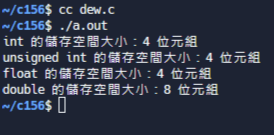
6.陳述
#include <stdio.h> /* 展示使用 goto 陳述句 */
int main()
{
int i, testvalue;
testvalue = 2;
for (i = 0; i < 10; i++)
{
if (testvalue == 2)
goto error;
}
printf("正常退出 for 迴圈\n");
error:
printf("testvalue 為 %d\n", testvalue);
}
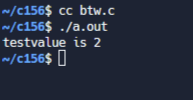
7.介於1-5的整數
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
/* switch 操作的範例 */
int main()
{
int this_is_a_number;
printf("請輸入介於1和5之間的整數:\n ");
scanf("%d", &this_is_a_number);
switch (this_is_a_number)
{
case 1:
printf("Case 1: 值為:%d", this_is_a_number);
break;
case 2:
printf("Case 2: 值為:%d", this_is_a_number);
break;
case 3:
printf("Case 3: 值為:%d", this_is_a_number);
break;
case 4:
printf("Case 4: 值為:%d", this_is_a_number);
break;
case 5:
printf("Case 5: 值為:%d", this_is_a_number);
break;
default:
printf("錯誤,值為:%d", this_is_a_number); /* 輸入的數字不在1到5之間 */
}
return 0;
}
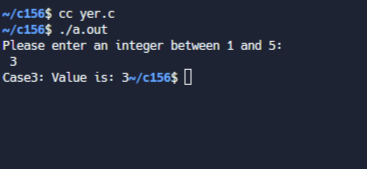
8.陣列
#include <stdio.h>
int main() {
int arr1[100][100]; /* 假設陣列大小不超過100x100 */
int i, j, k, l;
printf("請輸入陣列的行數和列數(以空格分隔):\n");
scanf("%d %d", &k, &l);
printf("請輸入陣列的元素:\n");
for (i = 0; i < k; i++) {
for (j = 0; j < l; j++) {
scanf("%d", &arr1[i][j]);
}
}
printf("您的陣列是:\n");
for (i = 0; i < k; i++) {
for (j = 0; j < l; j++) {
printf("%d ", arr1[i][j]);
}
printf("\n");
}
return 0;
}
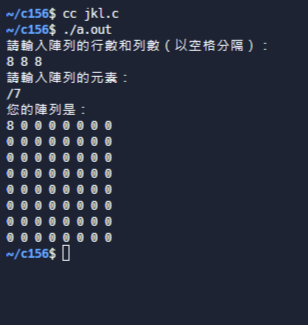
9.函數
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
/* 這段程式碼演示了一個函式的作用 */
/* 這個函式比較兩個數字並告訴哪一個更大 */
/* 使用者輸入三個數字,得知它們之間的大小關係! */
void myfunction(int a, int b); /* 函式及其參數的宣告 */
int first, second, third;
int main()
{
printf("請輸入第一個整數:");
scanf("%d", &first);
printf("請輸入第二個整數:");
scanf("%d", &second);
printf("請輸入第三個整數:");
scanf("%d", &third);
myfunction(first, second);
myfunction(first, third);
myfunction(second, third);
return 0;
}
void myfunction(int a, int b)
/* 這個函式在程式的 main{} 部分之外 */
/* 這個函式僅比較兩個參數 a 和 b,並告訴哪個更大 */
{
if (a > b)
printf("%d 大於 %d\n", a, b);
else if (a < b)
printf("%d 大於 %d\n", b, a);
else
printf("%d 和 %d 相等\n", a, b);
}
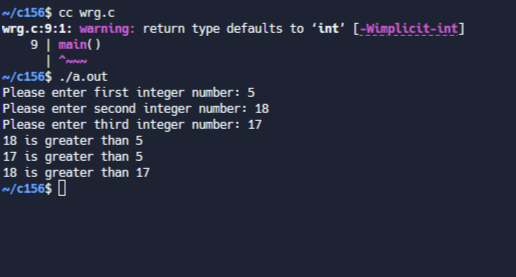
10.函式
/* 返回答案的函式 */
/* 找到學校一年級中成績最高的學生 */
#include <stdio.h>
double getmarks(double pupils[]);
int main()
{
double pupil;
/* 存放班級成績的數組在程式主體中預設 */
double marks[] = {10.6, 23.7, 67.9, 93.0, 64.2, 33.8, 57.5, 82.2, 50.7, 45.7};
/* 調用 getmarks 函式。該函式返回最高成績,然後將其存儲在 pupil 中 */
pupil = getmarks(marks);
printf("最高成績是 = %f", pupil);
return 0;
}
double getmarks(double pupils[])
{
int i;
double highest;
highest = 0;
/* 依次遍歷所有學生,存儲最高成績 */
for (i = 0; i < 10; ++i)
{
if (highest < pupils[i])
highest = pupils[i];
}
return highest; /* 將 highest 中的值返回到調用函式的地方 */
}
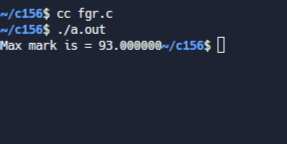
11.結構
/* 結構範例程式 */
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
/* 定義結構 */
struct Student {
int id;
char name[16];
double percent;
};
int main() {
/* 定義兩個屬於 "Student" 類型的數據位置 */
struct Student s1, s2;
/* 為 s1 結構賦值 */
s1.id = 56;
strcpy(s1.name, "Rob Smith");
s1.percent = 67.4;
/* 輸出結構 s1 */
printf("\nid:%d", s1.id);
printf("\n姓名:%s", s1.name);
printf("\n百分比:%lf", s1.percent);
/* 為 s2 結構賦值 */
s2.id = 73;
strcpy(s2.name, "Mary Gallagher");
s2.percent = 93.8;
/* 輸出結構 s2 */
printf("\nid:%d", s2.id);
printf("\n姓名:%s", s2.name);
printf("\n百分比:%l
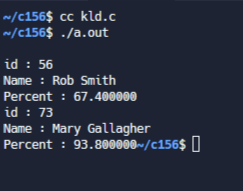
12.C語言
/* 這是我的第一個 C 語言程式 */
int main()
{
printf("我的第一個程式\n");
return 0;
}
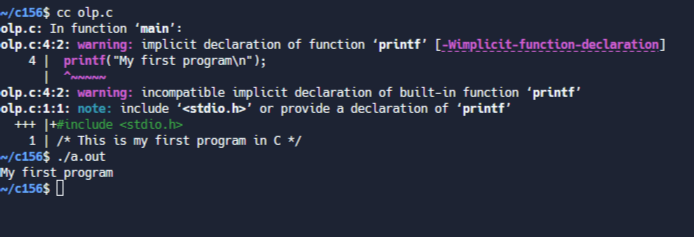
13.輸入、輸出
#include <stdio.h>
/* 讀取並顯示一個字符 */
int main() {
char c;
printf("輸入字符:");
c = getchar(); /* 讀取輸入的字符 */
printf("輸入的字符是:");
putchar(c); /* 輸出字符 */
return 0;
}
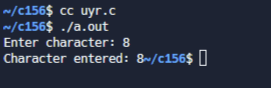
14.兩個數字的總和
/* 將兩個浮點數相加 */
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main() {
float this_is_a_number1, this_is_a_number2, total;
printf("請輸入一個數字:\n");
scanf("%f", &this_is_a_number1); /* 讀取十進制數字 */
printf("您輸入的數字為 %f\n", this_is_a_number1);
printf("請再輸入一個數字:\n");
scanf("%f", &this_is_a_number2); /* 讀取十進制數字 */
printf("您輸入的數字為 %f\n", this_is_a_number2);
total = this_is_a_number1 + this_is_a_number2; /* 將兩數相加 */
printf("總和為 %f\n", total);
return 0;
}
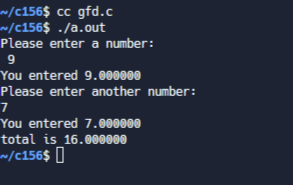
15.字元開關範例
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
/* 使用字元開關的範例 */
int main()
{
char this_is_a_character;
printf("請輸入字元 a、b、c、d 或 e:\n ");
scanf("%c", &this_is_a_character);
switch (this_is_a_character)
{
case 'a':
printf("輸入了 a");
break;
case 'b':
printf("輸入了 b");
break;
case 'c':
printf("輸入了 c");
break;
case 'd':
printf("輸入了 d");
break;
case 'e':
printf("輸入了 e");
break;
default:
printf("預設情況 ");
}
return 0;
}
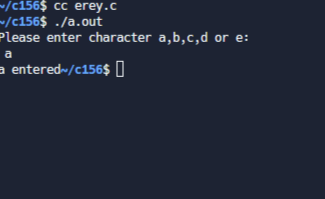
16.字串操作
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
/* 示範字串操作的程式 strlen、strcpy、strcat、strcmp */
int main() {
char borrow[7] = {'b', 'o', 'r', 'r', 'o', 'w', '\0'};
char string1[32] = "This is string1";
char string2[16] = "This is string2";
char string3[16];
int len;
/* 列印出字串的長度 */
len = strlen(string1);
printf("strlen(string1) : %d\n", len);
len = strlen(string2);
printf("strlen(string2) : %d\n", len);
len = strlen(string3);
printf("strlen(string3) : %d\n", len);
/* 將字串1複製到字串3中 */
strcpy(string3, string1);
printf("strcpy( string3, string1) : %s\n", string3);
len = strlen(string3);
printf("strlen(string3) after copy of string1 into string3 : %d\n", len);
/* 比較 string1 和 string3 (這些應該是相同的)*/
if (strcmp(string1, string3) == 0)
printf("字串相同\n");
/* 連接 string1 和 string2 */
strcat(string1, string2);
printf("strcat( string1, string2): %s\n", string1);
/* 連接後 string1 的總長度 */
len = strlen(string1);
printf("strlen(string1) after cat of string2
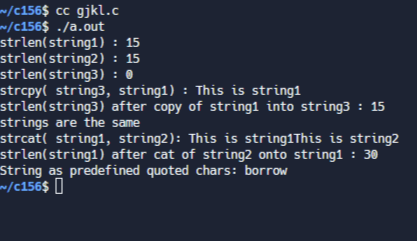
17.二維陣列
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
/* 二維數組測試範例 */
int main() {
int arr1[7][8];
int i, j, k, l;
printf("輸入行數和列數(最多 7 行最多 8 列)\n");
scanf("%d %d", &k, &l);
if (k > 7 || l > 8) {
printf("錯誤 — 行數最多為 7 列數最多為 8\n");
} else {
printf("輸入陣列\n");
for (i = 0; i < k; i++) {
for (j = 0; j < l; j++) {
scanf("%d", &arr1[i][j]);
}
}
printf("您的陣列是\n");
for (i = 0; i < k; i++) {
for (j = 0; j < l; j++) {
printf("%d ", arr1[i][j]);
}
printf("\n");
}
}
printf("陣列的第一行\n");
for (j = 0; j < l; j++) {
printf("%d ", arr1[0][j]);
}
printf("\n");
return 0;
}
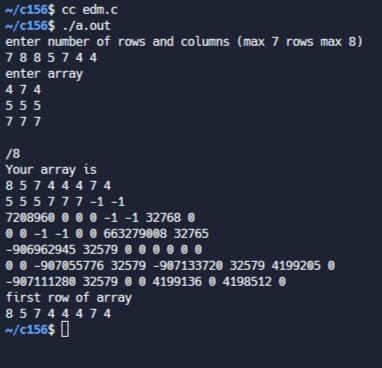
18.兩數相除
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
/* 兩個浮點數相除 */
int main() {
float this_is_a_number1, this_is_a_number2, total;
printf("請輸入一個數字:\n");
scanf("%f", &this_is_a_number1); /* 讀取數字 */
printf("您輸入了 %f\n", this_is_a_number1);
printf("請再輸入一個數字:\n ");
scanf("%f", &this_is_a_number2); /* 讀取數字 */
printf("您輸入了 %f\n", this_is_a_number2);
total = this_is_a_number1 / this_is_a_number2; /* 除以數字 */
printf("商為 %f\n", total);
return 0;
}
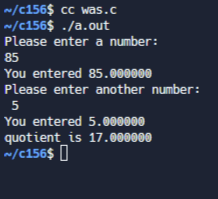
19.循環
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
/* 演示 do 循環 */
int main() {
float this_is_a_number, total;
int i;
total = 0;
i = 0;
/* do 循環循環直到 i 的值達到 10 */
do {
printf("請輸入一個數字:\n ");
scanf("%f", &this_is_a_number);
total = total + this_is_a_number;
i++;
} while (i < 10);
printf("總和為 %f\n", total);
return 0;
}
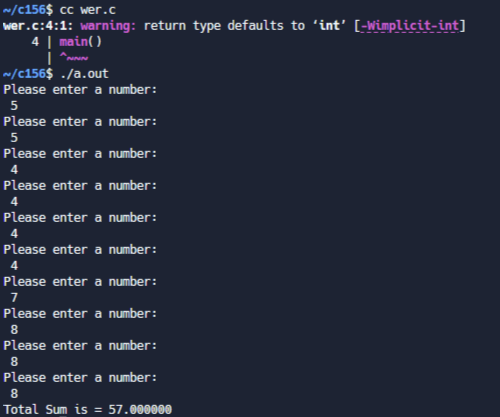
20.顯示字元程序
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
/* 顯示字元數組使用的程序 */
int main()
{
char arr2[10];/* 定義 10 個字元的數組 */
int i;
printf("enter 10 characters \n");
for (i = 0;i < 10;i++)
{
scanf("%c", &arr2[i]);
}
printf("Your 10 characters are \n");
for (i = 0;i < 10;i++)
{
printf("%c ", arr2[i]);
}
printf("\n");
}
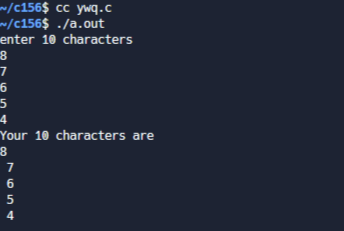
練習一 <<
Previous Next >> w11